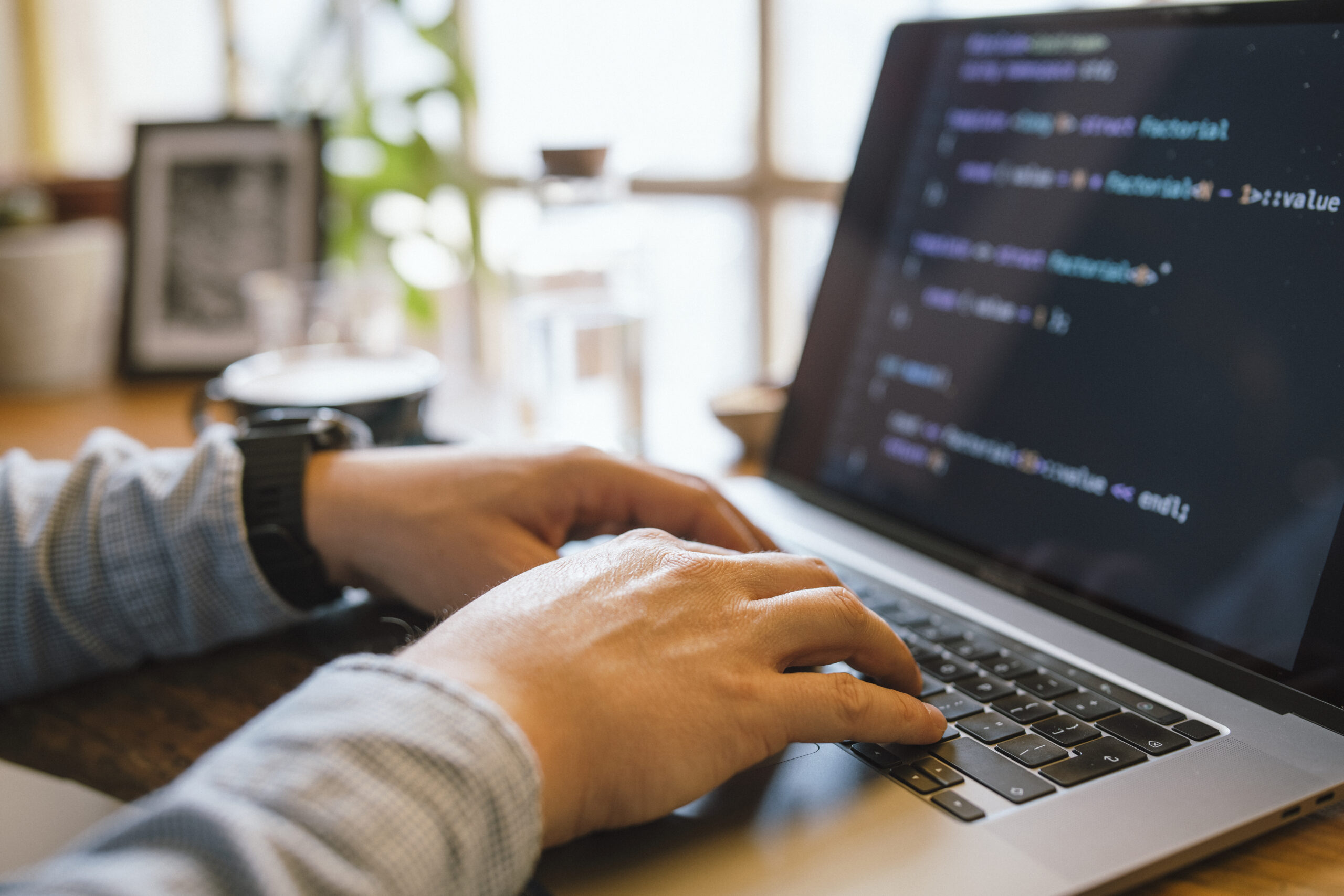
Debugging is Probably the most necessary — yet frequently neglected — expertise in a very developer’s toolkit. It isn't really pretty much correcting damaged code; it’s about comprehending how and why points go Completely wrong, and learning to Believe methodically to solve issues effectively. No matter if you are a rookie or maybe a seasoned developer, sharpening your debugging techniques can help you save several hours of frustration and dramatically improve your productivity. Here are quite a few procedures to assist builders amount up their debugging match by me, Gustavo Woltmann.
Grasp Your Resources
Among the quickest methods builders can elevate their debugging techniques is by mastering the instruments they use every single day. Although writing code is a single A part of development, recognizing the way to interact with it effectively throughout execution is Similarly critical. Modern day development environments occur Outfitted with potent debugging abilities — but a lot of developers only scratch the floor of what these resources can perform.
Just take, one example is, an Integrated Enhancement Setting (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These resources permit you to established breakpoints, inspect the value of variables at runtime, step by code line by line, and also modify code on the fly. When applied correctly, they Enable you to observe particularly how your code behaves for the duration of execution, which can be invaluable for tracking down elusive bugs.
Browser developer applications, which include Chrome DevTools, are indispensable for front-end builders. They let you inspect the DOM, check community requests, perspective true-time efficiency metrics, and debug JavaScript in the browser. Mastering the console, resources, and network tabs can change frustrating UI challenges into workable jobs.
For backend or program-level developers, equipment like GDB (GNU Debugger), Valgrind, or LLDB supply deep control in excess of running processes and memory management. Discovering these resources may have a steeper Understanding curve but pays off when debugging overall performance challenges, memory leaks, or segmentation faults.
Past your IDE or debugger, develop into cozy with Variation control devices like Git to understand code heritage, come across the exact second bugs ended up released, and isolate problematic modifications.
In the long run, mastering your tools suggests going over and above default configurations and shortcuts — it’s about creating an personal expertise in your progress atmosphere to ensure when challenges arise, you’re not misplaced in the dead of night. The greater you realize your resources, the more time it is possible to shell out resolving the particular issue rather then fumbling via the process.
Reproduce the trouble
One of the most critical — and often forgotten — ways in helpful debugging is reproducing the condition. Right before jumping into the code or producing guesses, builders need to have to make a regular setting or circumstance the place the bug reliably appears. With no reproducibility, repairing a bug gets to be a game of prospect, generally resulting in squandered time and fragile code changes.
The initial step in reproducing a difficulty is gathering just as much context as is possible. Check with thoughts like: What steps brought about the issue? Which ecosystem was it in — progress, staging, or manufacturing? Are there any logs, screenshots, or mistake messages? The more detail you have, the a lot easier it will become to isolate the exact conditions less than which the bug happens.
When you finally’ve collected enough facts, make an effort to recreate the problem in your neighborhood atmosphere. This could necessarily mean inputting precisely the same info, simulating identical consumer interactions, or mimicking system states. If The problem seems intermittently, look at creating automatic checks that replicate the edge scenarios or state transitions included. These exams don't just assist expose the situation but also avert regressions Down the road.
At times, the issue could be natural environment-specific — it would transpire only on certain working devices, browsers, or below distinct configurations. Applying tools like virtual devices, containerization (e.g., Docker), or cross-browser screening platforms is usually instrumental in replicating these kinds of bugs.
Reproducing the situation isn’t simply a stage — it’s a attitude. It calls for tolerance, observation, and a methodical method. But when you finally can consistently recreate the bug, you're currently halfway to fixing it. Using a reproducible circumstance, you can use your debugging tools much more efficiently, examination likely fixes safely and securely, and converse far more Evidently with your team or users. It turns an abstract criticism right into a concrete obstacle — Which’s where builders prosper.
Examine and Fully grasp the Mistake Messages
Mistake messages are sometimes the most valuable clues a developer has when something goes wrong. Rather than looking at them as disheartening interruptions, builders need to understand to take care of mistake messages as direct communications in the system. They often show you just what exactly took place, where by it took place, and at times even why it happened — if you know the way to interpret them.
Start out by looking through the message carefully and in comprehensive. A lot of developers, specially when beneath time stress, look at the primary line and straight away start off creating assumptions. But further from the error stack or logs might lie the real root trigger. Don’t just duplicate and paste error messages into search engines like yahoo — read and have an understanding of them initially.
Split the error down into pieces. Is it a syntax mistake, a runtime exception, or even a logic mistake? Does it issue to a certain file and line number? What module or operate activated it? These concerns can tutorial your investigation and stage you towards the responsible code.
It’s also valuable to understand the terminology on the programming language or framework you’re employing. Mistake messages in languages like Python, JavaScript, or Java usually observe predictable patterns, and Finding out to acknowledge these can significantly hasten your debugging procedure.
Some faults are vague or generic, and in Those people instances, it’s critical to look at the context by which the error occurred. Examine linked log entries, enter values, and recent adjustments from the codebase.
Don’t overlook compiler or linter warnings either. These normally precede bigger troubles and supply hints about opportunity bugs.
Ultimately, error messages are certainly not your enemies—they’re your guides. Discovering to interpret them properly turns chaos into clarity, serving to you pinpoint challenges quicker, minimize debugging time, and become a far more successful and self-assured developer.
Use Logging Sensibly
Logging is one of the most strong instruments in a very developer’s debugging toolkit. When made use of effectively, it offers real-time insights into how an software behaves, helping you realize what’s taking place beneath the hood with no need to pause execution or stage with the code line by line.
A great logging technique starts with understanding what to log and at what degree. Frequent logging amounts contain DEBUG, Information, WARN, Mistake, and Lethal. Use DEBUG for thorough diagnostic data all through enhancement, Details for normal functions (like productive commence-ups), WARN for opportunity difficulties that don’t crack the appliance, ERROR for actual complications, and Deadly once the system can’t go on.
Prevent flooding your logs with too much or irrelevant knowledge. A lot of logging can obscure important messages and decelerate your technique. Concentrate on key gatherings, condition adjustments, input/output values, and significant selection details with your code.
Format your log messages Plainly and constantly. Include context, for instance timestamps, request IDs, and performance names, so it’s simpler to trace issues in dispersed systems or multi-threaded environments. Structured logging (e.g., JSON logs) may make it even easier to parse and filter logs programmatically.
Through debugging, logs Allow you to track how variables evolve, what ailments are met, and what branches of logic are executed—all devoid of halting the program. They’re Specially valuable in generation environments where stepping by way of code isn’t achievable.
On top of that, use logging frameworks and resources (like Log4j, Winston, or Python’s logging module) that guidance log rotation, filtering, and integration with monitoring dashboards.
Eventually, intelligent logging is about balance and clarity. That has a well-imagined-out logging method, it is possible to lessen the time it will take to identify challenges, acquire deeper visibility into your purposes, and Increase the General maintainability and trustworthiness of one's code.
Consider Similar to a Detective
Debugging is not merely a technical activity—it is a method of investigation. To effectively recognize and fix bugs, developers need to technique the procedure similar to a detective resolving a mystery. This state of mind aids stop working complex problems into manageable elements and comply with clues logically to uncover the foundation induce.
Start by gathering evidence. Look at the indicators of the situation: mistake messages, incorrect output, or effectiveness challenges. Identical to a detective surveys against the law scene, obtain just as much applicable information and facts as you'll be able to without having jumping to conclusions. Use logs, check instances, and user reviews to piece together a clear photograph of what’s going on.
Next, form hypotheses. Ask yourself: What can be producing this actions? Have any improvements just lately been manufactured for the codebase? Has this situation transpired prior to under identical situation? The purpose is always to narrow down possibilities and establish likely culprits.
Then, take a look at your theories systematically. Try and recreate the trouble in a managed setting. In the event you suspect a selected purpose or part, isolate it and confirm if The problem persists. Like a detective conducting interviews, talk to your code inquiries and let the effects direct you closer to the reality.
Spend shut focus to modest particulars. Bugs often cover inside the least predicted locations—similar to a missing semicolon, an off-by-a person mistake, or possibly a race problem. Be thorough and client, resisting the urge to patch the issue devoid of totally being familiar with it. Momentary fixes might disguise the real issue, just for it to resurface later.
And lastly, maintain notes on Anything you experimented with and learned. Just as detectives log their investigations, documenting your debugging system can preserve time for future challenges and aid Many others realize your reasoning.
By imagining like a detective, developers can sharpen their analytical competencies, strategy challenges methodically, and turn out to be simpler at uncovering hidden troubles in elaborate methods.
Publish Checks
Writing exams is one of the best ways to boost your debugging techniques and All round growth performance. Checks not just aid capture bugs early but will also function a security net that gives you assurance when creating adjustments to the codebase. A very well-analyzed software is much easier to debug mainly because it allows you to pinpoint specifically in which and when a difficulty takes place.
Get started with device checks, which deal with unique capabilities or modules. These compact, isolated tests can quickly expose whether a specific piece of logic is Operating as anticipated. Whenever a test fails, you immediately know where to look, significantly reducing some time expended debugging. Device assessments are Specially valuable for catching regression bugs—concerns that reappear right after previously remaining fastened.
Upcoming, integrate integration tests and end-to-close assessments into your workflow. These assistance be sure that a variety of elements of your software get the job done collectively smoothly. They’re significantly practical for catching bugs that arise in intricate methods with numerous factors or companies interacting. If one thing breaks, your checks can inform you which Portion of the pipeline unsuccessful and beneath what conditions.
Producing exams also forces you to definitely Feel critically about your code. To test a aspect appropriately, you'll need to be familiar with its inputs, predicted outputs, and edge circumstances. This volume of comprehension Normally sales opportunities to better code framework and less bugs.
When debugging an issue, composing a failing exam that reproduces the bug could be a robust first step. When the check fails continually, you are able to target correcting the bug and observe your take a look at move when The difficulty is resolved. This strategy makes sure that the same bug doesn’t return Later on.
Briefly, writing exams turns debugging from the disheartening guessing sport into a structured and predictable process—aiding you catch additional bugs, a lot quicker and much more reliably.
Just take Breaks
When debugging a tough difficulty, it’s simple to become immersed in the challenge—observing your monitor for several hours, trying Resolution just after solution. But Probably the most underrated debugging equipment is just stepping away. Getting breaks will help you reset your head, lower irritation, and infrequently see The difficulty from the new perspective.
When you're as well close to the code for as well lengthy, cognitive fatigue sets in. You may commence overlooking apparent mistakes or misreading code which you wrote just hours earlier. In this state, your brain gets to be much less efficient at problem-resolving. A brief stroll, a coffee break, or simply switching to another undertaking for ten–15 minutes can refresh your concentrate. Several developers report finding the foundation of a challenge once they've taken time for you to disconnect, letting their subconscious work during the qualifications.
Breaks also support avoid burnout, Particularly during extended debugging classes. Sitting in front of a monitor, mentally caught, is read more not only unproductive but will also draining. Stepping absent enables you to return with renewed energy and also a clearer frame of mind. You may instantly detect a missing semicolon, a logic flaw, or simply a misplaced variable that eluded you right before.
In case you’re stuck, a fantastic rule of thumb is to set a timer—debug actively for 45–sixty minutes, then take a five–ten minute crack. Use that time to maneuver close to, stretch, or do a little something unrelated to code. It might experience counterintuitive, Specially under restricted deadlines, but it in fact leads to speedier and more effective debugging In the long term.
In brief, getting breaks is not a sign of weakness—it’s a wise system. It provides your Mind House to breathe, improves your point of view, and helps you stay away from the tunnel eyesight That always blocks your progress. Debugging is often a psychological puzzle, and rest is a component of resolving it.
Learn From Each individual Bug
Each bug you face is a lot more than simply a temporary setback—It truly is a possibility to grow as being a developer. No matter whether it’s a syntax mistake, a logic flaw, or a deep architectural concern, each can train you a little something valuable when you take the time to reflect and evaluate what went Mistaken.
Start out by inquiring you a few important queries when the bug is solved: What brought about it? Why did it go unnoticed? Could it are caught before with improved tactics like device testing, code critiques, or logging? The answers frequently reveal blind spots in your workflow or comprehending and assist you to Develop stronger coding routines shifting ahead.
Documenting bugs will also be a wonderful pattern. Continue to keep a developer journal or retain a log in which you Take note down bugs you’ve encountered, the way you solved them, and That which you uncovered. After a while, you’ll start to see patterns—recurring challenges or popular faults—which you could proactively keep away from.
In group environments, sharing what you've learned from the bug using your peers can be Primarily powerful. Irrespective of whether it’s via a Slack message, a brief produce-up, or a quick knowledge-sharing session, serving to Other folks avoid the exact challenge boosts group performance and cultivates a more robust Studying society.
Far more importantly, viewing bugs as lessons shifts your way of thinking from annoyance to curiosity. As opposed to dreading bugs, you’ll start appreciating them as vital elements of your progress journey. In any case, some of the ideal developers will not be the ones who publish perfect code, but individuals who continuously study from their mistakes.
In the long run, each bug you correct provides a fresh layer for your talent set. So upcoming time you squash a bug, have a second to replicate—you’ll come away a smarter, extra capable developer on account of it.
Summary
Enhancing your debugging techniques requires time, follow, and tolerance — however the payoff is big. It will make you a more effective, confident, and capable developer. The following time you happen to be knee-deep inside of a mysterious bug, keep in mind: debugging isn’t a chore — it’s a chance to be improved at Anything you do.